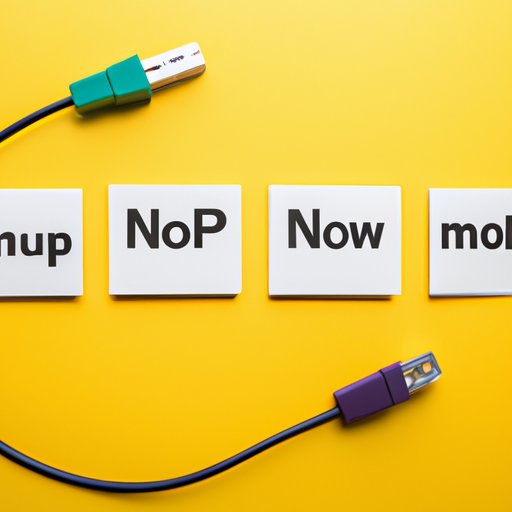
I. Introduction
If you’re starting out with Node.js, you’ll soon come across npm, the default package manager that comes with Node.js. npm is a powerful tool that enables developers to easily install, manage, and share code packages and libraries. Whether you’re building a simple web app or a complex enterprise solution, npm provides an extensive library of packages and modules that can help simplify your development process.
Installing npm is an essential part of getting started with Node.js, as it provides the necessary tools to manage your code dependencies. This comprehensive guide takes you step-by-step through the process of installing npm on Windows, Mac, and Linux, as well as troubleshooting common errors and best practices for updating and managing npm packages.
II. Installing npm on Windows
Installing npm on Windows can be a bit tricky, as there are a few dependencies you’ll need to install before getting started. Here is a step-by-step guide to help you:
- Download and install Node.js from the official website.
- Open the command prompt or PowerShell as an administrator.
- Run the following command to install npm:
npm install npm@latest -g
- Verify that npm has been installed by running the following command:
npm --version
If you encounter any errors during the installation process, check out our troubleshooting guide below.
III. Installing npm on Mac
Installing npm on Mac is relatively straightforward, as most of the dependencies are already included with the operating system. Here’s how to do it:
- Download and install Node.js from the official website.
- Open the Terminal.
- Run the following command to install npm:
npm install npm@latest -g
- Verify that npm has been installed by running the following command:
npm --version
If you encounter any errors during the installation process, check out our troubleshooting guide below.
IV. Installing npm on Linux
Installing npm on Linux varies depending on your distribution and package manager. However, the general process is similar across most distributions.
Here’s a step-by-step guide:
- Use your distribution’s package manager to install Node.js.
- Open the Terminal.
- Run the following command to install npm:
sudo npm install npm@latest -g
- Verify that npm has been installed by running the following command:
npm --version
If you encounter any errors during the installation process, check out our troubleshooting guide below.
V. Installing the latest version of npm using Node Version Manager (NVM)
Node Version Manager (NVM) is a popular tool that enables developers to manage multiple versions of Node.js and npm on the same machine. Here’s how you can install the latest version of npm using NVM:
A. Explanation of NVM
NVM allows you to easily switch between different versions of Node.js, which is useful when working on projects that require specific versions of Node.js or npm. With NVM, you can install multiple versions of Node.js and npm side-by-side, and switch between them as needed.
B. Step-by-Step Guide
- Install NVM by following the instructions on the official website.
- Open the Terminal.
- Use NVM to install the latest version of Node.js:
nvm install node
- Use NVM to install the latest version of npm:
nvm install-latest-npm
- Verify that npm has been installed by running the following command:
npm --version
If you encounter any errors during the installation process, check out our troubleshooting guide below.
VI. Simplified Instructions for Beginners on Installing and Using npm
A. Explanation of Basic Concepts
Before we dive into the installation process for npm, it’s important to understand some basic concepts:
- Node.js: a JavaScript runtime environment that allows you to run JavaScript on the server-side.
- npm: a package manager that allows you to install, manage, and share code packages and libraries.
- Package: a collection of files that contain code, assets, and metadata needed to fulfill a specific function.
- Dependency: a package that another package relies on to function.
B. Step-by-Step Guide
- Download and install Node.js from the official website.
- Open the Terminal or Command Prompt.
- Run the following command to install npm:
npm install npm@latest -g
- Verify that npm has been installed by running the following command:
npm --version
If you encounter any errors during the installation process or are having trouble using npm, check out our troubleshooting guide below.
VII. Using Command Line to Install npm: A Beginner’s Guide
A. Explanation of Command Line
The command line is a powerful tool that allows you to interact with your computer using text-based input. By using the command line, you can perform a wide range of tasks quickly and efficiently.
B. Step-by-Step Guide
- Open the Terminal or Command Prompt.
- Run the following command to install Node.js:
sudo apt-get install nodejs
- Run the following command to install npm:
sudo apt-get install npm
- Verify that npm has been installed by running the following command:
npm --version
If you encounter any errors during the installation process or are having trouble using npm, check out our troubleshooting guide below.
VIII. How to Troubleshoot Common Errors When Installing npm on Your Machine
A. Explanation of Common Errors
The installation process for npm can sometimes encounter errors, whether you’re installing on Windows, Mac, Linux or using command line. Some common errors include:
- Permission errors: npm requires administrative access to install packages, so you may encounter errors related to permissions or Windows UAC.
- Network errors: if you’re behind a firewall or using a proxy, npm may not be able to access the internet.
- Dependency conflicts: some packages may require specific versions of other packages, which can cause conflicts during installation.
- File system errors: npm stores packages and dependencies on the file system, which can sometimes cause issues with file permissions or path length.
B. Step-by-Step Guide for Troubleshooting
Here are some common solutions to common errors when installing npm:
- Permission errors: run the command prompt or PowerShell as an administrator; use the “sudo” command (for Mac and Linux).
- Network errors: check your internet connection, firewall settings, and proxy settings.
- Dependency conflicts: use the “npm dedupe” command to resolve conflicts; use the “npm update” command to update dependencies to their latest versions.
- File system errors: use the “npm cache clean” command to clear the cache; check that the file path is not too long.
C. Best Practices for Avoiding Errors
To avoid issues during installation, it’s best to follow these best practices:
- Only install packages from trusted sources.
- Regularly update Node.js and npm to their latest versions.
- Check package dependencies to avoid version conflicts.
- Update and uninstall packages regularly to avoid unnecessary clutter.
- Use a version control system, such as Git, to manage your code and changes.
IX. Installing and Managing npm Dependencies for Your Node.js Project
A. Explanation of Dependencies
When building a project with Node.js, you’ll likely need to use external packages or libraries to help you complete certain functions. These external packages are referred to as dependencies, and they can be installed and managed using npm.
The package.json file is used to manage dependencies for a Node.js project, and it provides a detailed list of the dependencies used by your project. Package.json also makes it easy to share your project with others, as they can simply run “npm install” to download and install all the necessary dependencies for the project.
B. Step-by-Step Guide for Installation and Management
- Open the Terminal or Command Prompt.
- Navigate to the directory where your project is located.
- Run the following command to create a package.json file:
npm init
- Follow the on-screen prompts to set up the package.json file.
- Once you’ve set up the package.json file, you can install dependencies by running the following command:
npm install <package-name> --save
- You can also install and save multiple dependencies at once by running:
npm install <package1-name> <package2-name> --save
- Verify that the dependencies have been installed by checking the package.json file.
- To uninstall a dependency, run the following command:
npm uninstall <package-name> --save
C. Best Practices for Managing Dependencies
To manage dependencies effectively, you should follow these best practices:
- Update dependencies regularly to the latest version.
- Avoid installing unnecessary dependencies.
- Use semantic versioning to manage version conflicts.
- Use Git or another version control system to track changes to your dependencies.
X. Best Practices for Updating and Uninstalling npm Packages
A. Explanation of Updating and Uninstalling Packages
When you have installed packages via npm, it’s essential to keep them updated and remove them when they are no longer needed. This helps keep your project healthy and free from unnecessary clutter.
B. Step-by-Step Guide for Updating and Uninstalling
- Open the Terminal or Command Prompt.
- To update an individual package, run the following command:
npm update <package-name>
- To update all packages, run the following command:
npm update
- To uninstall an individual package, run the following command:
npm uninstall <package-name>
- To uninstall all packages, run the following command:
npm uninstall --save-dev
C. Best Practices for Managing Packages
To manage packages effectively, you should follow these best practices:
- Update packages regularly to the latest version.
- Remove unused packages from your project.
- Use peer dependencies to avoid conflicts.
- Use semantic versioning to manage version conflicts.
- Use Git or another version control system to track changes to your packages.
XI. Conclusion
In this comprehensive guide, we’ve covered everything you need to know about installing npm on Windows, Mac, and Linux. We’ve also provided troubleshooting tips for common installation errors, as well as best practices for managing dependencies and packages.
Whether you’re a beginner or an experienced developer, npm is an essential tool for any project that uses Node.js. By following the steps in this guide, you’ll be well on your way to using npm to its full potential.
Additional Resources for Further Learning
- Official npm documentation: https://docs.npmjs.com/
- Node.js official website: https://nodejs.org/en/
- NVM official website: https://github.