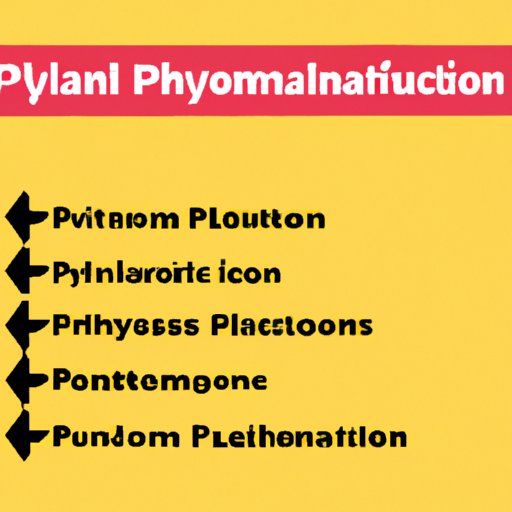
I. Introduction
Python functions are reusable blocks of code that perform specific tasks. They help to improve code readability, reduce redundancy, and make programming more efficient. However, calling a function in Python can be tricky, especially for beginners.
In this comprehensive guide, we will explore different ways to call a function in Python. We will cover everything from defining and calling functions within the same script to using modules and lambda functions. This guide is intended for beginners who are new to Python programming.
II. Defining and Calling a Function in the Same Script
A Python function is defined using the “def” keyword followed by the function name and parentheses. Any arguments that the function takes are placed within the parentheses, separated by commas. The function body is indented and can contain any valid Python code.
To call a function in the same script, place the function call after the function definition. Python will execute the function call and then continue with the rest of the script.
Here’s an example:
def greet(name):
print("Hello, " + name + "!")
greet("John") # prints "Hello, John!"
In this example, we define a function called “greet” that takes one argument, “name”. The function body prints a personalized greeting using the name argument.
We then call the “greet” function with the argument “John”. Python executes the function call and prints “Hello, John!” to the console.
III. Calling a Function from Another Function
Python allows us to nest functions, which means we can define a function inside another function. When we call the outer function, it will execute the inner function as well.
Here’s an example:
def outer():
def inner():
print("Inner function executed!")
print("Outer function executed!")
inner()
outer() # prints "Outer function executed!" and "Inner function executed!"
In this example, we define an outer function that has an inner function nested inside it. The inner function simply prints a message to the console.
When we call the outer function, it executes the print statement within the function body and then calls the inner function, which also prints to the console.
IV. Using the Input Function to Execute a Function
The input() function in Python allows us to prompt the user to enter input, which we can then use to execute a specific function.
Here’s an example:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
return a / b
operation = input("Choose an operation (+, -, *, /): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if operation == '+':
result = add(num1, num2)
elif operation == '-':
result = subtract(num1, num2)
elif operation == '*':
result = multiply(num1, num2)
elif operation == '/':
result = divide(num1, num2)
print(result)
In this example, we define four functions for basic arithmetic operations: add, subtract, multiply, and divide. We then use the input() function to prompt the user to choose an operation and enter two numbers.
Based on the user’s input, we call the corresponding function and pass in the user’s input as arguments. The function returns the result, which we then print to the console.
V. Using Command-Line Arguments to Call a Function
Command-line arguments in Python allow us to pass parameters and execute a function based on user input from the command line.
Here’s an example:
import sys
def greet(name):
print("Hello, " + name + "!")
if len(sys.argv) > 1:
greet(sys.argv[1])
In this example, we import the “sys” module, which provides access to some variables used or maintained by the interpreter and to functions that interact strongly with the interpreter. We then define a “greet” function that takes one argument, “name”, and prints a personalized greeting.
Next, we check if the length of the “sys.argv” list is greater than 1. If it is, we call the “greet” function with the first command-line argument as the name parameter.
To run this script from the command line and pass in a command-line argument, we can enter the following command:
python script.py John
This will execute the script and pass “John” as the command-line argument, which will be used as the “name” parameter for the “greet” function.
VI. Using a Module to Call a Function
A module in Python is a file containing Python definitions and statements. We can import modules into other scripts and use their functions in our code.
Here’s an example:
# module.py
def greet(name):
print("Hello, " + name + "!")
# script.py
import module
module.greet("John") # prints "Hello, John!"
In this example, we define a “greet” function in a separate module called “module.py”. We then import the “module” module into another script and call the “greet” function from the module.
VII. Using a Lambda Function
A lambda function in Python is a small anonymous function that can take any number of arguments but can only have one expression. Lambda functions are often used as a shortcut for simple operations that don’t require a full function definition.
Here’s an example:
def multiply(n):
return lambda x: x * n
double = multiply(2)
print(double(5)) # prints 10
In this example, we define a “multiply” function that takes one argument, “n”. The function returns a lambda function that multiplies its argument by “n”.
We then call the “multiply” function with an argument of 2 and assign the result to a variable called “double”. Finally, we call “double” with an argument of 5, which returns 10.
VIII. Using the exec() Function
The exec() function in Python allows us to execute dynamic Python code at runtime. However, using exec() can be risky as it can execute potentially harmful code and lead to security vulnerabilities.
Here’s an example:
code = 'print("Hello, World!")'
exec(code) # prints "Hello, World!"
In this example, we define a string variable containing a simple Python program that prints “Hello, World!” to the console. We then pass the string variable to the exec() function, which executes the program and prints the message to the console.
IX. Conclusion
In this comprehensive guide, we explored different ways to call a function in Python, from defining and calling functions within the same script to using modules and lambda functions. We also explained the potential security vulnerabilities associated with using the exec() function.
We hope this guide has helped you understand the fundamentals of calling functions in Python. We encourage you to apply these concepts to your own Python programs and continue learning more about Python programming.