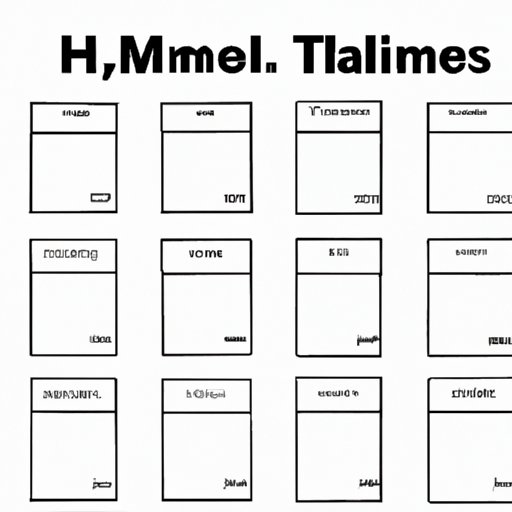
Introduction
If you have ever designed a website using HTML and CSS, you have probably come across the need to center a div element. A div is a container that allows you to group and organize the contents of your webpage. Despite its simple purpose, centering a div can be a bit challenging, especially for beginners. In this article, we will discuss various ways of centering a div, including using CSS margin property, Flexbox, CSS grid, position property, JavaScript, and frameworks/libraries.
Using CSS margin property
The margin property in CSS defines the space outside the border of an element. By setting the left and right margins of the div to ‘auto,’ we can center it horizontally.
Here is a step-by-step guide on how to center a div using the margin property:
Step 1: Open a new HTML document and create a new div with a class of “center”.
Step 2: In the style section of your document, define the width and height of the div element, along with its margins.
“`css
.center {
width: 50%;
height: 50px;
margin: auto;
}
“`
Step 3: Save your stylesheet and preview your HTML document. You should see the centered div element.
Using Flexbox
The Flexbox layout is a powerful CSS tool for building flexible and responsive layouts. Flexbox allows you to easily center elements both horizontally and vertically by setting the ‘justify-content’ and ‘align-items’ properties to ‘center.’
Here is a step-by-step guide on how to center a div element using Flexbox:
Step 1: Open a new HTML document and create a new div with a class of “wrapper.”
“`html
“`
Step 2: In the style section of your document, define the wrapper’s display as a Flexbox layout and set its alignment properties.
“`css
.wrapper {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
“`
Step 3: Define the width and height of the child (centered) element within the wrapper.
“`css
.center {
width: 50%;
height: 50px;
}
“`
Using CSS Grid
CSS Grid layout offers a more robust and advanced way of creating grid-based website layouts. CSS Grid allows for precise control and flexibility when it comes to positioning and aligning HTML elements.
Here is a step-by-step guide on how to center a div element using CSS Grid:
Step 1: Open a new HTML document and create a new div element with a class of “wrapper.”
“`html
“`
Step 2: In the style section of your document, declare the display property of the wrapper element as “grid”. Define its grid template and set the “place-items” property to “center.”
“`css
.wrapper {
display: grid;
place-items: center;
height: 100vh;
grid-template-columns: repeat(12, 1fr);
grid-template-rows: repeat(12, 1fr);
}
“`
Step 3: Define the width and height of the centered element within the wrapper.
“`css
.center {
width: 50%;
height: 50px;
}
“`
Using position property
The position property allows you to position elements anywhere on the page and offers several options, including absolute, relative, and fixed positioning. Using the ‘position’ and ‘left’ and ‘right’ properties together allows you to center a div element horizontally.
Here is a step-by-step guide on how to center a div element using the position property:
Step 1: Open a new HTML document and create a new div element with a class of “center”.
“`html
“`
Step 2: In the style section of your document, define the width and height of the div element, along with the ‘position’ property set to ‘absolute.’
“`css
.center {
width: 50%;
height: 50px;
position: absolute;
left: 0;
right: 0;
margin: auto;
}
“`
Using JavaScript
JavaScript is a powerful programming language used for creating dynamic websites. It allows for complex interactions between the user and the web page. In this case, JavaScript can be used to center a div element dynamically, based on the available screen size.
Here is a step-by-step guide on how to center a div element using JavaScript:
Step 1: Open a new HTML document and create a new div element with a class of “center”.
“`html
“`
Step 2: In the JavaScript section of your document, create a function to center the div element.
“`javascript
function centerDiv() {
var div = document.querySelector(“.center”);
div.style.position = “absolute”;
div.style.top = “50%”;
div.style.left = “50%”;
div.style.transform = “translate(-50%, -50%)”;
}
window.onload = centerDiv;
“`
Using a Framework/Library
Frameworks and libraries like Bootstrap and Foundation offer pre-built templates and stylesheets for building websites quickly and efficiently. They provide classes and utilities for centering div elements, making it easier to build responsive and functional websites.
Here is an overview of how to center a div element using popular frameworks and libraries:
Bootstrap:
“`html
“`
Foundation:
“`html
“`
Conclusion
In conclusion, there are various ways to center a div element in HTML and CSS, as demonstrated in this article. The CSS margin property, Flexbox, CSS Grid, position property, JavaScript, and frameworks/libraries all offer unique solutions for centering div elements. While there is no single “best” method, choosing the most appropriate one for your specific use case will depend on the requirements of your website.
For more information on how to center div elements, check out the W3C documentation and online tutorials provided by leading web development communities. Remember, practice makes perfect, so don’t hesitate to experiment and try different methods until you find the one that works for you.