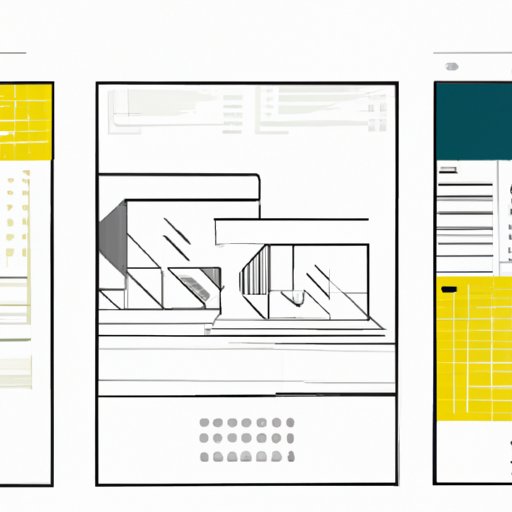
Introduction
Have you ever tried to center an image on your HTML page only to find it stubbornly staying to the left or right of the screen? It can be frustrating to have an image that doesn’t align with the rest of your content. Knowing how to center images in HTML is an essential skill for any web developer or designer, as it helps to create visually appealing and professional-looking layouts.
Using CSS to Center Images
CSS stands for Cascading Style Sheets, and it is a language used to describe how an HTML document should look. It is a powerful tool that can be used to style all aspects of a web page, including images.
Setting Margins to Auto
The easiest way to center an image using CSS is to set the margins to auto. This works by automatically adjusting the margins around the image so that it is centered within its container. Simply add the following code to any CSS selector that targets your image:
img { display: block; margin: auto; }
The “display:block” property ensures that the image takes up the full width of its container, making it easier to center.
Using Table Tags
HTML table tags can be used to create a grid layout on a website. They can also be used to center images on a page.
Inserting the image into a table
To center an image within a cell of a table, simply insert the image tag inside a td tag and use the “align” attribute to specify that the image should be centered. Here is an example:
![]() |
The “align” attribute is set to “center” which tells the image to center horizontally within the table cell.
Using Flexbox
Flexbox is a layout mode in CSS which allows you to arrange elements in a flexible way. It provides an efficient way to center images without the need for any margins or tables.
Including “justify-content” and “align-items” properties
The “justify-content” property is used to align items horizontally, while the “align-items” property is used to align items vertically. To center an image using flexbox, start by creating a container element which contains the image. Use the following code to center the image, assuming the image is in a div tag with a class of “image-container”:
.image-container { display: flex; justify-content: center; align-items: center; }
This centers the image both horizontally and vertically within the container.
Using Bootstrap
Bootstrap is a popular front-end framework that includes many pre-made components like buttons, forms, and navigation menus. It also has a set of classes that can be used to center images.
Adding Classes to Specify Alignment
You can use the “mx-auto” class in combination with the “d-block” class to center an image. The “mx-auto” class sets the horizontal margin to “auto”, while the “d-block” class sets the display property to “block”. Here is an example:
This centers the image both horizontally and vertically within its container.
Using JavaScript
Javascript is a versatile language that can be used for many aspects of web development, including centering images. By calculating the dimensions of the image, you can then position the image at the center of its container.
Calculating Dimensions of the Image
To center an image with JavaScript, start by selecting the image element using the “getElementById” method. Next, use the “clientWidth” and “clientHeight” properties to calculate the width and height of the image. Finally, use the “style” property to set the “margin-left” and “margin-top” properties to half of the calculated dimensions. Here is an example:
var img = document.getElementById("center-image"); img.style.marginLeft = -(img.clientWidth/2) + "px"; img.style.marginTop = -(img.clientHeight/2) + "px";
The above code centers the image with an id of “center-image” both horizontally and vertically within its container.
Using Inline Styles
Inline styles are a way to apply CSS styles directly to an HTML element. While not the most efficient way to style a web page, they can be used to quickly center images without the need for additional CSS or JavaScript.
Including a Style Attribute in the Image Tag
To center an image with inline styles, simply add a “style” attribute to the image tag and use the “margin” property to center it. Here is an example:
The “display: block” property ensures that the image takes up the full width of its container, while the “margin: auto” property centers it both horizontally and vertically.
Conclusion
Many methods exist for centering images within HTML. The most appropriate technique will depend on the project and the audience.
Summary of the Different Techniques to Center Images in HTML
- Using CSS to set margins to auto
- Using HTML table tags to align an image within a table cell
- Using flexbox to center an image horizontally and vertically within its container
- Using Bootstrap classes to quickly center an image
- Using JavaScript to calculate dimensions and position an image
- Using inline styles for a quick way to center an image
Reminder that the Style Should Match the Needs of the Project and Audience
When choosing a method for centering an image, it is important to consider the needs and preferences of the project and audience. For example, some projects may require a responsive layout, while others may require a more traditional grid system.
Encouragement to Practice and Experiment with Different Styles
Remember that the more you use these techniques, the more comfortable you will become with centering images in HTML. Follow best practices and experiment with different styles to find the best solution for your project.