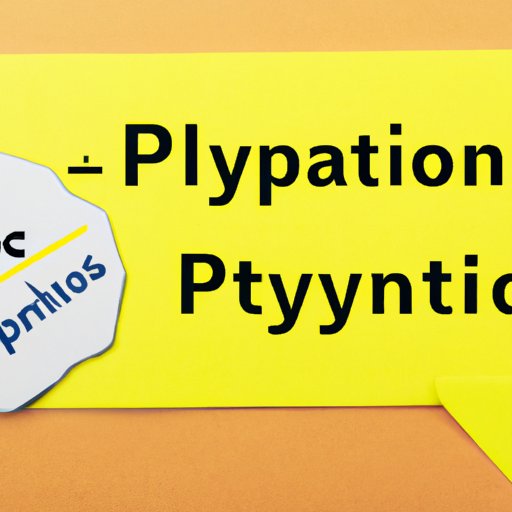
I. Introduction
Python is a powerful and widely-used programming language known for its simplicity and efficiency. However, as with any programming language, it can be challenging to read and understand code without proper documentation. This is where comments come in. Comments are lines of text within your code that are not executed but provide valuable information about the purpose, functionality, and usage of your code.
In this article, we will guide you through the process of writing effective comments in Python. We will explore the different types of comments, their use cases, and best practices for commenting code. We will also introduce you to python docstrings, a powerful tool for documenting code. Finally, we will discuss using comments in Python for debugging and collaboration.
II. How to Write Comments in Python
Before we dive into the different types of comments, let’s define what comments are and their purpose in Python. Comments are non-executable lines in your code that are meant to provide information, explanations, or clarifications about the code. Comments begin with a # symbol and can appear anywhere in the code. Although comments are not part of the program execution, they are important for understanding the code and its functionality.
Let’s take a closer look at the different types of comments in Python:
Single-line comments
Single-line comments begin with the # symbol and are used to add comments to a single line of code. This type of comment should be used to clarify or explain a particular line of code. For example:
x = 5 # assign the value 5 to x
Multi-line comments
Multi-line comments are used to add comments to multiple lines of code. They begin and end with three quotes (”’), known as triple quotes or docstrings. Multi-line comments are often used to add a description of the code, explain the purpose of the function, or provide examples of how to use the code. For example:
''' This function returns the result of adding two numbers. Parameters: num1 (float): The first number num2 (float): The second number Returns: (float): The sum of num1 and num2 ''' def add_numbers(num1, num2): return num1 + num2
Inline comments
Inline comments are used to add comments to the same line of code as the actual statement. These comments begin with the # symbol and are used to explain or clarify the code on that line. Inline comments should be used sparingly and only when the line of code is not self-explanatory. For example:
x = 5 # assign the value 5 to x (this is redundant and unnecessary)
It is important to use the appropriate type of comment for the situation to make your code easier to read and understand.
III. Best Practices for Commenting in Python
Now that we know how to write comments in Python, let’s discuss why comments are important and what information should be included in a comment. Comments are an important part of code documentation as they can aid in understanding the code’s functionality, purpose, limitations, and certain assumptions made. In short, comments can enhance code readability and maintainability.
When writing comments in Python, it is essential to keep the following best practices in mind:
Explain what the code does
Comments should clearly explain what the code does. The reader should be able to understand the code’s function and role within the codebase from the comment. This can be achieved through the use of descriptive variable and function names, as well as outlining the algorithm and its intended procedure where applicable. For example:
# calculate the area of a triangle using base and height def calculate_triangle_area(base, height): return 0.5 * (base * height)
Explain why the code is needed
Comments should also explain why the code is necessary. This can help other developers understand the context behind the code and how it fits into the broader system, its use cases and limitations where appropriate. For example:
# this function verifies user information before granting access to the application def verify_user(user_id, password): ...
Include any assumptions or constraints on the code
Comments can also provide information about any assumptions or constraints on the code. This can help other developers understand how the code works in current systems and any compatibility issues that may exist. For example:
# this function is compatible with only Python3 and above
Write clear and concise comments
Comments should be clear and concise. Avoid writing unnecessarily long comments where a shorter explanation suffices. If multi-line comments are used, ensure they are well-formatted and correctly indented to ensure legibility. Use simple and easy-to-understand language, especially for multi-line comments. For example:
''' This function returns the result of adding two numbers. '''
IV. Documenting Code with Python Docstrings
Python docstrings are special form of comments that are used to document functions, modules and classes. Docstrings are included in the code and provide information about an object (i.e. function, module or class), its purpose, and any arguments that the object takes. Unlike traditional Python comments, docstrings have a specific format and can be accessed programmatically via a class or function’s __doc__ attribute.
There are two types of docstrings – single-line and multi-line docstrings. A single-line docstring is used to provide a brief summary of a class or function and should appear on the first line of the function or class. Multi-line docstrings, on the other hand, provide a more detailed explanation and can span multiple lines or paragraphs. Multi-line docstrings are enclosed in triple quotes and are ideal for explaining the function’s usage and functionality, as well as method signatures and examples of use.
Here is an example of a multi-line docstring:
def calculate_triangle_area(base, height): """ This function calculates the area of a triangle. Parameters: base (float): The length of the base of the triangle. height (float): The height of the triangle. Returns: (float): The area of the triangle. """ return 0.5 * (base * height)
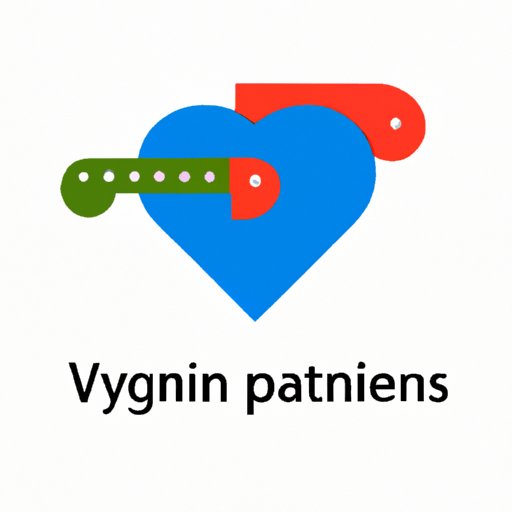
V. Using Comments in Python for Debugging
Comments can be an extremely useful tool when debugging Python code. Debugging comments are used to identify and track down errors or unexpected behavior in the code. When writing debugging comments, it is important to be specific and clear about the problem and its location. This can help other developers replicate the situation and test possible solutions to the problem.
Here is an example of a debugging comment:
if x <= 0: # error: x must be greater than zero ...
In addition to highlighting errors and bugs, debugging comments can help track the cause of errors in code, for example:
# step 1: fetch data from the database # step 2: validate the data against a defined schema # step 3: transform the data to a common format # step 4: store the data into a NoSQL database data = fetch_data() if validate_data(data): transformed_data = transform_data(data) store_data(transformed_data) else: # error: data validation failed ...
VI. Writing Clean and Concise Comments in Python
Clean and concise comments can greatly improve the readability and maintainability of your code. Here are some tips for writing comments that are easy to read and understand:
Use proper grammar and spelling
Like all written communication, your comments should use proper grammar and spelling. Poorly written comments can be confusing and detract from the clarity of the code.
Format comments for readability
Make sure your comments are well-formatted and easy to read. Use indentation, line breaks, and formatting where appropriate. Use whitespace to separate comments from code blocks and to make your code more readable.
Avoid unclear or unnecessary comments
Avoid redundant or unclear comments that do not add any value to the understanding of the code. For example, avoid commenting on trivial steps or code blocks that are self-explanatory.
VII. Using Comments in Python for Collaboration
Collaboration is an important aspect of software development. Good commenting practices can greatly enhance a team's ability to work together effectively. Here are some tips for writing collaborative comments:
Be mindful of your readers
Keep in mind who will be reading your comments. Write comments that are easily understandable for your target audience. Keep your comments technical but avoid using jargon or acronyms that are specific to your organization or team.
Use clear and concise language
When writing collaborative comments, keep your language clear and concise. Avoid lengthy or complex explanations, and use simple words and phrases to describe your code and its functionality.
Stay organized with consistent commenting styles
Develop and maintain a consistent style across your codebase. This can help make your comments more readable and understandable, and makes it easier to collaborate as a team.
VIII. Conclusion
Comments are an essential aspect of Python programming. They are used to document code, aid in debugging, and facilitate collaboration between team members. In this article, we have covered the different types of comments in Python, as well as best practices for writing clean, concise and effective comments. We have also explored Python docstrings and how they can be used to document code.
By using comments effectively, you can make your code more readable, understandable and maintainable, ensuring your code is efficient and scalable for any future changes.