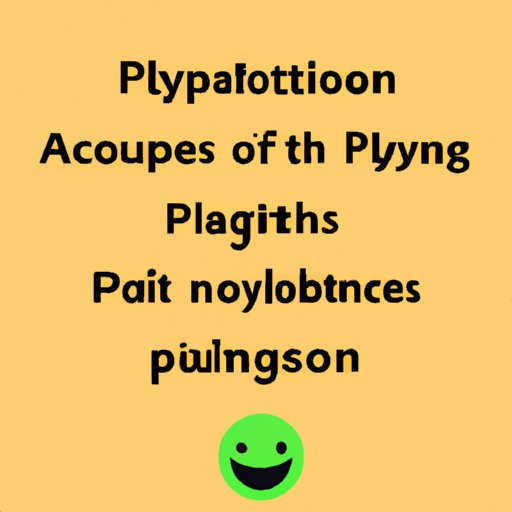
I. Introduction
If you’re a Python programmer, you know how important it is to write clean, well-documented code. One key aspect of this is commenting out chunks of code when they aren’t needed or are causing issues. In this article, we’ll explore the art of commenting out multiple lines in Python, including multiple techniques for doing so, common mistakes to avoid, and some expert tips for streamlining this process and using it to your advantage.
II. “Mastering the Art of Commenting Out Multiple Lines in Python: A Step-by-Step Guide”
To begin, let’s define what we mean by “commenting out.” Essentially, commenting out code is a way of temporarily disabling it without deleting it altogether. This can be useful when debugging, testing, or simply experimenting with different approaches to a problem.
There are three primary ways to comment out multiple lines in Python, each with its own advantages and drawbacks:
Method 1: Using ‘#’ to Comment Out Each Line Individually
This method involves placing a ‘#’ symbol at the beginning of each line you want to comment out. While simple to implement, it can be time-consuming and cumbersome when dealing with large blocks of code.
Here’s an example of what this would look like in practice:
# This is a comment
# This is another comment
# And another!
Method 2: Using Triple Quotes to Enclose and Comment Out Multiple Lines
This method involves using triple quotes to enclose and comment-out a block of code all at once. This can be quicker and more efficient than method 1, especially if you’re dealing with a lot of code at once.
Here’s what this would look like in code:
"""
This entire block of code is now commented out
See how easy that was?
"""
Method 3: Using a Keyboard Shortcut to Comment Out Multiple Lines
Method 3 is perhaps the most efficient approach, as it involves using a keyboard shortcut to comment-out entire blocks of code at once. While this method requires a bit of setup, it can be a lifesaver for programmers who frequently need to comment-out large chunks of code.
To use this method, you’ll need to create a custom keyboard shortcut that inserts the necessary comment characters. Here’s an example shortcut you could use:
ctrl + alt + c
With this shortcut set up, you can easily select a block of code and hit the shortcut to comment it out all at once.
To set up this shortcut, consult your code editor’s documentation for information on how to create custom keyboard shortcuts. This will vary from editor to editor.
III. “5 Techniques to Comment Out Multiple Lines in Python: Which One Works Best for You?”
Now that we’ve explored the three primary methods for commenting out multiple lines in Python, let’s take a closer look at some additional techniques.
Technique 1: Use the ‘if False’ Statement to Comment Out Multiple Lines
This method involves wrapping an entire block of code in an ‘if False’ statement, effectively commenting it out. This can be useful when you want to keep the commented-out code visible in your editor, but need to suppress its execution during testing or debugging.
Here’s an example of how this would work:
if False:
print("This line is commented out")
print("So is this one!")
Technique 2: Using Conditional Statements to Control Commenting-Out
This method involves using conditional statements to control when chunks of code are commented-out. This can be useful when debugging, as it allows you to quickly comment-out code when certain conditions are met.
Here’s an example of how this would work:
if debugging_enabled:
# This entire block of code will execute if debugging_enabled is True
print("Debugging enabled!")
do_debugging()
else:
# This entire block of code will execute if debugging_enabled is False
do_normal_stuff()
Technique 3: Use String Concatenation to Disable Chunks of Code
This technique involves disabling chunks of code by concatenating them into a string, effectively commenting them out in the process. This can be useful when you need to quickly disable certain sections of your code during testing or debugging.
Here’s an example of how this would work:
code_to_disable = '''
This chunk of code is now commented out
See how easy that was?
'''
# To disable the code, simply don't execute the code_to_disable variable:
# code_to_disable
Technique 4: Use a Code Toggle to Enable/Disable Blocks of Code
This method involves using a code toggle, such as a global variable, to enable/disable chunks of code. This can be useful when you need to quickly switch between different configurations or testing scenarios.
Here’s an example of how this would work:
enable_foo = True
if enable_foo:
# This entire block of code will execute if enable_foo is True
do_foo_stuff()
else:
# This entire block of code will execute if enable_foo is False
do_bar_stuff()
Technique 5: Use a Custom Python Script to Comment Out Code Automatically
This technique involves using a custom Python script to automatically comment-out code based on certain criteria. This can be useful when you need to quickly comment-out large amounts of code, or for automating certain parts of your workflow.
Here’s an example of how this would work:
# This script will comment-out all lines containing the word 'debug' in a given file
with open('myfile.py', 'r') as f:
contents = f.readlines()
new_contents = []
for line in contents:
if 'debug' in line:
new_line = "#" + line
else:
new_line = line
new_contents.append(new_line)
with open('myfile.py', 'w') as f:
f.writelines(new_contents)
When executed, this script will comment-out all lines containing the word ‘debug’ in a file called ‘myfile.py’.
IV. “Expert Tips to Speed up Your Python Coding: Commenting Out Multiple Lines like a Pro”
Now that we’ve covered several methods for commenting-out multiple lines in Python, let’s explore some tips for streamlining this process and using it to your advantage.
Tip 1: Use Keyboard Shortcuts to Speed Up the Process
As we mentioned earlier, using keyboard shortcuts can be a great way to speed up the process of commenting out large chunks of code. Take the time to create custom shortcuts for your preferred comment-out method, and you’ll save time and effort in the long run.
Tip 2: Use Comments to Document Your Thought Process
While commenting out code can be useful for testing and debugging, it can also be a helpful tool when documenting your thought process. This is especially true when working on large, complex projects with many collaborators.
By commenting-out different approaches to a problem, or explaining why certain lines of code were disabled, you can help others understand your reasoning and thought process. This can lead to more efficient code development, fewer mistakes, and better team collaboration.
Tip 3: Experiment with Different Comment-Out Methods to Find Your Preferred Approach
While we’ve presented several different approaches to commenting-out code in Python, there’s no one “right” way to do it. Experiment with different methods and techniques to find the approach that works best for you and your workflow.
Tip 4: Don’t Over-Comment Your Code
While commenting-out code can be a helpful tool, it’s important not to overdo it. Too many comments can clutter up your code and make it difficult to read and follow.
When commenting-out code, focus on explaining why you’re disabling it and how it fits into your larger code development strategy. Avoid unnecessary comments and aim for clarity and simplicity.
V. “Common Mistakes to Avoid When Commenting Out Multiple Lines in Python”
While commenting-out can be a valuable tool for Python programmers, there are a few common mistakes to avoid:
Mistake 1: Commenting-Out Too Much Code
As we mentioned earlier, it’s important not to over-comment your code. Commenting-out large chunks of code can make your code difficult to read and follow, and can also cause confusion when working with other team members.
When deciding which lines of code to comment-out, focus on those that are causing issues or are no longer needed. Avoid the temptation to comment-out entire blocks of code unless necessary.
Mistake 2: Forgetting to Uncomment Code When You’re Done Testing or Debugging
One common mistake is forgetting to uncomment code when you’re done testing or debugging. This can cause the code to behave unexpectedly, and can cause issues when working with other team members.
When commenting-out code, make sure to also add comments that explain why the code is commented-out and when it should be uncommented. This will help ensure that you remember to uncomment the code when you’re done.
Mistake 3: Not Using Conditional Statements to Better Control Commenting-Out
While commenting-out code can be a useful tool, it’s important to not rely too heavily on this technique. Instead, consider using conditional statements or other approaches to better control when and why code is disabled.
By using conditional statements, you can more easily enable/disable certain code blocks based on specific conditions. This can help you quickly switch between different testing or debugging scenarios, without needing to rely on commenting-out code excessively.
VI. “Debugging in Python: How to Use Comments to Isolate Issues in Your Code”
Finally, it’s worth noting that commenting-out code can be a helpful tool when debugging in Python.
By selectively commenting-out different parts of your code, you can isolate issues and get a better understanding of how your code is behaving. For example, you might comment-out a section of code to see if a specific error goes away, or to test a different approach to solving a problem.
Experienced programmers often use commenting-out as a tool to streamline the debugging process and get to the root of issues more quickly. By experimenting with different approaches to commenting-out and leveraging this technique to your advantage, you can become a more efficient and effective Python programmer.
VII. Conclusion
In conclusion, mastering the art of commenting-out multiple lines in Python is an important skill for any programmer. Whether you’re testing, debugging, or simply experimenting with different approaches to a problem, commenting-out code can save you time and effort.
In this article, we’ve explored several different methods for commenting-out code, including keyboard shortcuts, conditional statements, and more. We’ve also offered tips and guidance for streamlining the process and avoiding common mistakes.
We encourage readers to experiment with these techniques and find the approach that works best for them. By leveraging commenting-out as a tool to isolate issues and streamline the debugging process, you can become a more productive and efficient Python programmer.