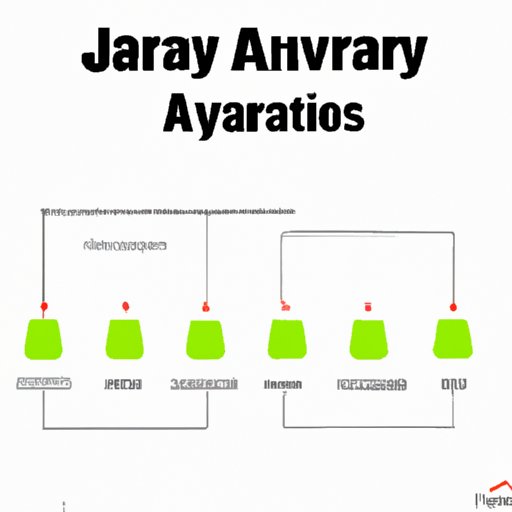
I. Introduction to Java Arrays: Learn How to Declare an Array and Its Uses
If you’re just getting started with Java programming, you’ll quickly discover the importance of arrays. Arrays are a powerful tool for grouping similar types of data together, making it easier to manage and manipulate data within your program. But how do you declare an array in Java, and what are the key things you need to know?
A. What is an Array?
In computer science, an array is a data structure that stores a collection of similar items in contiguous memory locations. Put simply, it’s a way of grouping multiple values of the same type together, which makes it easier to read, write, and manipulate the data. Arrays are widely used in programming languages like Java, where they’re a staple feature of many applications.
B. How is an Array Used in Java?
Arrays are used extensively in Java programming to store and manipulate collections of data. For example, you might use an array to store a list of numbers, a group of employee names, or a set of key-value pairs. In essence, an array provides a simple, efficient way to store and manage data that belongs together in a logical sense.
C. Why is it Important to Learn Array Declaration in Java?
As a Java programmer, learning how to declare an array is a crucial step in your development. Arrays are one of the most important data structures in Java, and mastering their use can significantly improve the performance and efficiency of your code.
II. Getting Familiar with Java Arrays: How to Declare an Array Step-by-Step
Before we dive into the syntax of array declaration in Java, let’s take a moment to go over the basic steps you need to follow to declare an array.
A. Steps for Declaring an Array in Java
Declaring an array in Java involves three steps:
- Determine the size of the array.
- Choose the data type for the array.
- Write the syntax for array declaration.
B. Examples of Array Declaration Using Different Data Types
The syntax for declaring an array in Java depends on the data type you’re working with. Here are some examples of array declaration using different data types:
int[] myArray = new int[10];
String[] myArray = new String[5];
double[] myArray = new double[20];
III. Java Array Syntax: Basic and Advanced Techniques for Array Declaration
A. Basic Syntax for Array Declaration in Java
At its core, the syntax for array declaration in Java is relatively simple. Here’s the basic syntax:
dataType[] arrayName = new dataType[arraySize];
For example:
int[] myArray = new int[10];
This declares an integer array named myArray
with a size of 10 elements.
B. Advanced Techniques for Array Declaration in Java
Once you have a basic understanding of array declaration in Java, you can start experimenting with more advanced techniques. Here are a few examples:
1. Initializing an Array During Declaration
Instead of creating an empty array and then populating it with data, you can initialize an array during declaration. Here’s an example:
String[] myArray = {"apple", "orange", "banana", "grape"};
This declares and initializes a string array named myArray
with four elements.
2. Creating a 2D Array
A 2D array is simply an array of arrays. Here’s an example:
int[][] myArray = new int[2][3];
This declares a 2D integer array named myArray
with two rows and three columns.
3. Creating an Array of Objects
In Java, you can create an array of objects as easily as you would create an array of primitives. Here’s an example:
Person[] myArray = new Person[5];
This creates an empty array of Person
objects with a length of five.
IV. Understanding Array Declaration in Java: Simple Techniques for Beginners
A. Common Mistakes Made in Array Declaration in Java
When you’re just starting out with Java programming, it’s easy to make mistakes in your array declaration. Here are a few common mistakes to watch out for:
- Forgetting to specify the data type
- Specifying the data type incorrectly
- Using an invalid array size
- Using an uninitialized array
B. Tips for Avoiding Errors in Array Declaration
To avoid errors in your array declaration, it’s important to pay attention to detail and follow best practices. Here are a few tips:
- Always specify the data type for your array
- Double-check that your data type is correct
- Choose a valid array size
- Initialize your arrays before using them
C. Common Pitfalls and How to Avoid Them
One common pitfall of array declaration in Java is dealing with out-of-bounds errors. To avoid these errors, you should always test your arrays before attempting to access their elements. Additionally, it’s a good idea to use methods like array.length
to make sure you don’t accidentally go beyond the bounds of your array.
V. A Beginner’s Guide to Declaring Arrays in Java: Tips and Tricks
A. Tips for Optimizing Array Declaration in Java
As you become more comfortable with array declaration in Java, you can start to think about optimizing your code. Here are a few tips for optimizing your array declarations:
- Avoid using arrays that are too large for your needs
- Use the correct data type to minimize memory usage
- Consider using dynamic arrays if you need to add or remove elements frequently
- Use initialization shortcuts to simplify your code
B. How to Check for Array Size Limitations
When declaring arrays in Java, it’s important to be aware of size limitations. The maximum size of an array in Java is determined by the amount of memory your program is allowed to use. To check for size limitations, you can use the Runtime.maxMemory()
method, which returns the maximum amount of memory that your program can allocate.
C. Best Practices for Creating and Declaring Arrays in Java
Some best practices for creating and declaring arrays in Java include using sensible variable names, using data types appropriate to your needs, and initializing your arrays before using them. Additionally, you should try to avoid using arrays in situations where other data structures like lists or maps might be more appropriate.
VI. Mastering Arrays in Java: A Comprehensive Guide to Array Declaration
A. Advanced Topics Related to Array Declaration in Java
If you’ve mastered the basics of array declaration in Java, there are several advanced topics you can explore. Here are a few examples:
1. Implementing Dynamic Arrays
Dynamic arrays are arrays that can resize themselves as needed. In Java, you can implement dynamic arrays using classes like ArrayList
or Vector
.
2. Working with Multi-Dimensional Arrays
Multi-dimensional arrays are arrays of arrays. In Java, you can create 2D, 3D, or even higher dimensional arrays using simple syntax.
3. Built-In Array Methods in Java
Java provides several built-in methods that you can use to manipulate and work with arrays. These methods include Arrays.sort()
, Arrays.toString()
, and Arrays.copyOf()
.
B. Expert-Level Examples for Array Declaration
For expert-level examples of array declaration in Java, try exploring open-source Java projects on platforms like GitHub. Reading through other people’s code can give you insights into advanced techniques and best practices you might not have considered before.
VII. Top Tips for Declaring Arrays in Java: Expert Techniques for Programmers
A. Advanced Methods for Initializing and Populating Arrays
One advanced technique for initializing arrays in Java is to use loops or other algorithms to populate the array with values. For example, you might use a loop to populate an array with the first 100 prime numbers.
B. How to Use Arrays in Multi-Threaded Applications
When using arrays in multi-threaded applications, it’s important to ensure that your code is thread-safe. This might involve using synchronization or other techniques to prevent race conditions or other concurrency issues.
C. Tips and Tricks for Optimizing and Managing Large Arrays
If you’re working with large arrays in Java, there are several tips and tricks you can use to optimize your code. Some of these include using iterative algorithms instead of recursive ones, using parallel processing techniques, and splitting your arrays into smaller, more manageable pieces.
Conclusion
Declaring arrays in Java is a fundamental skill for any programmer. By understanding the basic syntax and techniques for creating and declaring arrays, you can start to build more efficient and powerful applications. Whether you’re just getting started with Java programming or you’re an experienced developer looking to optimize your code, the tips and tricks in this article will help you master the art of array declaration in Java.