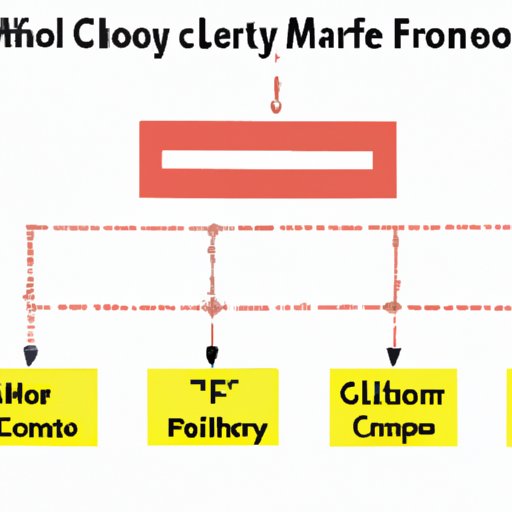
Introduction
As a C programmer, understanding how to manage memory allocated for arrays is crucial. Failure to properly free memory can result in serious consequences, including memory leaks and poor system performance. This article aims to provide a comprehensive guide on how to free an array in C, including step-by-step instructions and best practices.
Syntax and Definition of Arrays in C
Arrays are an essential aspect of C programming, providing a way to store and access multiple values of the same data type. In C, an array is defined as a collection of similar data types under a single name. For example, an array of integers can be defined as follows:
int numbers[5];
This code creates an integer array named “numbers” with 5 elements. Array elements are accessed using index numbers, starting with 0. For example:
numbers[0] = 1;
This code assigns the value 1 to the first element in the “numbers” array.
Dynamically Allocating Memory for an Array using `malloc()`
In C, arrays can be statically or dynamically allocated. When an array is statically allocated, the compiler reserves a fixed amount of memory for the array during compilation. However, when an array is dynamically allocated, memory is allocated while the program is running.
The `malloc()` function is used in C to dynamically allocate memory. The function takes one argument, which is the number of bytes of memory needed. For example, to allocate memory for an array of 5 integers:
int *arr = (int*)malloc(5 * sizeof(int));
This code allocates memory for 5 integers and returns a pointer to the first element in the array. The `(int*)` typecasting is used to convert the pointer returned by `malloc()` to the appropriate data type.
Why it is Crucial to Free Memory Allocated for an Array
When memory is dynamically allocated in C, it is the programmer’s responsibility to free the memory when it is no longer needed. Failure to do so can lead to memory leaks, which can cause serious issues, including poor system performance, crashes, and security vulnerabilities.
Freeing memory that has been allocated for an array is particularly important in scenarios where the array is allocated multiple times. Forgetting to free previously allocated memory can lead to memory leaks and ultimately, system failure.
How to Free an Array in C using `free()` Function
The `free()` function in C is used to release memory that was previously allocated with `malloc()` or `calloc()`. To free an array allocated with `malloc()`, simply call the `free()` function and pass it the pointer to the allocated memory.
For example:
int *arr = (int*)malloc(5 * sizeof(int)); // allocate memory for array
*…code to use the array…*
free(arr); // free memory allocated for array
This code dynamically allocated an array, used it, and then freed the memory allocated for it.
Tips for Avoiding Memory Leaks When Working with Arrays
To avoid memory leaks when working with arrays in C, it is essential to follow best practices for memory management. Here are some tips:
Ensure you only free memory that has been allocated
Attempting to free memory that has not been allocated can cause the program to crash. Always ensure that you are only freeing memory that has been dynamically allocated.
Use a debugger to identify memory leaks
Debuggers are powerful tools that can help identify memory leaks and other issues. Use a debugger to identify and fix any memory management issues in your program.
Conclusion
Managing memory is an essential aspect of C programming. Failure to properly free memory allocated for arrays can lead to serious consequences, including memory leaks and poor system performance. By using the `malloc()` and `free()` functions correctly and following best practices for memory management, you can avoid these issues and optimize system performance.