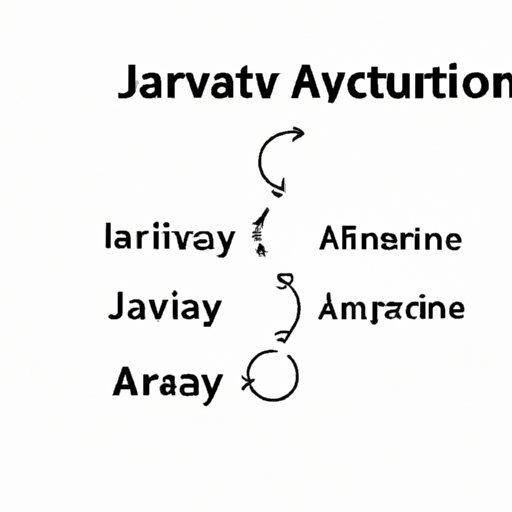
I. Introduction
Arrays are an essential part of programming, and Java provides multiple methods to initialize them. This article aims to explore different ways to initialize an array in Java and identify the pros and cons of each method. But first, let’s define what an array is in Java, why initialization is crucial, and the purpose of the article.
A. Definition of an array in Java
An array is a data structure consisting of a collection of elements, each identified by an index or a key. In Java, arrays are objects that can store fixed-sized sequential collections of elements of the same type. An array contains primitives, such as int, char, or boolean, or objects such as String or custom classes.
B. Importance of initializing an array
Java requires arrays to initialize before use explicitly. Failing to initialize an array could result in runtime errors, null pointer exceptions, and unpredictable program behaviors. Initializing an array sets the initial values in each element of the array, so when you access them, you can get a predictable value.
C. Purpose of the article
This article aims to provide a comprehensive guide on different ways of initializing an array in Java. We’ll explore the step-by-step guide, use case examples, comparison of methods, best practices, and FAQs to help you become proficient in initializing arrays.
II. Step-by-step guide to initializing an array in Java
Java provides different methods to initialize an array; let’s explore them one by one.
A. Using a for loop
A for loop is a popular way of initializing an array programmatically. Here’s how we can initialize an array using a for loop:
1. Code example
// Initialize an array of integers from 1 to 5 using for loop
int[] numbers = new int[5];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i + 1;
}
2. Explanation of code
The code creates a new integer array with a size of 5 and sets a loop variable (i) to zero. The for loop runs until the loop variable, i, is less than the length of the numbers array. Inside the loop, we set the current index of the numbers array to the value of i plus one. In each iteration, we increment i by one until the loop terminates.
3. Pros and cons
Pros:
- Programmatically set values in the array
- Customizable for different types of arrays or values
Cons:
- Inefficient for large arrays
- More prone to errors
B. Using arrays.fill()
The java.util.Arrays.fill() method allows you to initialize an entire array with a given value.
1. Code example
// Initialize an array of strings with the same value
String[] names = new String[3];
Arrays.fill(names, "John");
2. Explanation of code
The code creates a new array of strings with a size of 3. The code then uses the java.util.Arrays.fill() method to initialize each element in the array with the string “John.”
3. Pros and cons
Pros:
- Shorter code
- Useful for initializing arrays with the same value or null
Cons:
- Cannot initialize an array with variable values
- Not flexible for different types of arrays or values
C. Using static initialization
You can declare and initialize an array in a single line of code using static initialization.
1. Code example
// Initialize an array of integers using static initialization
int[] numbers = {1, 2, 3, 4, 5};
2. Explanation of code
The code declares and initializes an array of integers using the static keyword. The values are declared inside curly braces separated by commas.
3. Pros and cons
Pros:
- Simplicity and readability
- Static initialization avoids errors
Cons:
- Cannot initialize an array with variable values
- It is less flexible for complex data types or structures
III. Use case example of initializing an array in Java
A. Real-life scenario
Suppose you want to create a program that calculates the sum of the first n positive integers. Here, we need to initialize an array of integers from 1 to n inclusive.
B. Coding example
import java.util.Scanner;
public class SumOfPositiveInt {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a positive integer: ");
int n = sc.nextInt();
int[] numbers = new int[n];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i + 1;
}
int sum = 0;
for (int i : numbers) {
sum += i;
}
System.out.println("The sum of the first " + n + " positive integers is " + sum);
}
}
C. Implementation example
The program prompts a user to enter a positive integer n. It then creates a new integer array with a size of n using a for loop. It sets each index in the array with the values from 1 to n inclusive. It then calculates the sum of the integers using a for-each loop and outputs the result.
IV. Comparison of different methods for initializing an array in Java
Each method has its advantages and disadvantages, depending on the situation. Let’s compare these methods based on their speed, simplicity, and readability.
A. Speed comparison
The speed of initializing an array varies depending on the number of elements, the size of the elements, and the method used. A for loop is the slowest method because it requires a loop to initialize every element.
Explanation
The for loop initializes each element of an array one at a time, so as the number of elements increases, the time required to initialize the array also increases proportionately. The arrays.fill() method and static initialization methods are faster because they initialize all the elements of an array at once.
Pros and cons
Pros:
- Static initialization and the arrays.fill() methods are faster than the for loop method
- The for loop method can be faster when initializing arrays with a small number of elements (less than 100)
Cons:
- The speed of initialization varies depending on the size and number of elements
- Initialization speed may not be significant, depending on its usage
B. Simplicity comparison
Simplicity and ease of use are crucial for writing quality code. The static initialization and arrays.fill() are easy to use because they require fewer lines of code.
Explanation
The static initialization method requires only one line of code, and the elements are declared and initialized instantly. The arrays.fill() method requires two lines of code, and all elements in an array are initialized with a single value. However, the for loop method requires a loop to initialize every element, which can be complex for larger arrays and prone to errors.
Pros and cons
Pros:
- Static initialization is the simplest method
- The fill() method is easier than a for loop because it has fewer lines of code
Cons:
- For loop method can be more complex for larger arrays
- Static initialization and the fill() method may not be suitable for initializing complex arrays or structures
C. Readability comparison
Readability is key for understanding, testing, and debugging code. The static initialization method is more readable because the values are declared on a single line.
Explanation
Static initialization provides a concise and readable way of initializing an array. In this method, the values are declared on a single line, which can make it easier to read and maintain.
Pros and cons
Pros:
- Static initialization provides readable and concise code
- It is easy to spot syntax errors or typos in static initialization by reviewing a single line
Cons:
- Static initialization may not be suitable for initializing more complex data structures.
D. Conclusion on the best method to use
The best method for initializing an array depends on various factors. Static initialization is the best method for initializing arrays with a small number of elements, while fill() is suitable for initializing arrays of the same value. A for loop is useful, especially when you need to initialize an array with variable data or complex structures.
V. Best practices for initializing an array in Java
To write quality code that is maintainable, readable, and easy to debug, it is essential to follow common best practices when initializing arrays.
A. Avoiding magic numbers
Avoid using numbers directly in code as it makes the code less readable and harder to maintain. Instead, use constants or variables to represent numbers.
B. Avoiding over-complexity
Avoid complexity when initializing arrays. Use simple initialization methods that make the code more readable and easier to maintain.
C. Maintaining code readability
When initializing arrays, ensure that the code is easy to understand and readable. Avoid including too many lines of code in a single method to make the code more maintainable.
D. Example of a correct way to initialize an array of objects
public class Car {
private int licensePlate;
private String make;
...
}
// Create an array of Car objects
Car[] cars = new Car[]{
new Car(12345, "Ford"),
new Car(67890, "Toyota"),
new Car(24680, "Honda")
};
VI. FAQs on initializing an array in Java
Here are some frequently asked questions about initializing an array in Java:
A. What is the syntax for initializing an array?
The syntax for initializing an array is as follows:
datatype[] arrayname = {values}
B. Can I initialize an array with a mix of data types?
You can only initialize an array with a single data type. If you need to store multiple data types in an array, use an object array.
C. Can I change the size of an initialized array?
No, you cannot change the size of an initialized array. You need to create a new array and copy the old data into the new array to resize an array.
D. What is the difference between static and dynamic initialization?
The difference can be explained as follows:
- Static initialization involves declaring and initializing an array in a single statement.
- Dynamic initialization involves initializing an array dynamically, by setting its values in code.
VII. Conclusion
In conclusion, initializing arrays correctly and efficiently is essential in Java programming. In this article, we have explored different methods of initializing an array in Java, including the for loop, arrays.fill(), and static initialization. We have also provided a use case example, a comparison of initializing array methods, and best practices for initializing an array. By following the best practices and techniques we have discussed in this article, you can write code that is concise, readable, and easy to maintain.