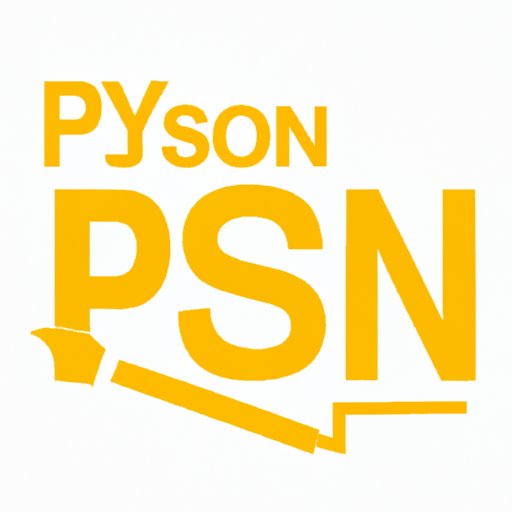
Introduction
If you’ve ever tried to open a JSON file, you know that it isn’t as simple as just double-clicking on it. JSON files are often used to store and exchange data, and are becoming more and more popular in today’s technology-driven world. But how do you open a JSON file? In this article, we’ll explore the different ways to open a JSON file, as well as the best tools and techniques for working with them.
5 Simple Steps to Open JSON Files on Your Computer
The first step in working with JSON files is to know how to open them. Here are five simple steps to follow:
- Open your web browser or text editor
- Go to the file menu and select “Open”
- Navigate to the location of your JSON file and select it
- Click “Open”
- Explore the contents of your JSON file!
It really is that simple! Here’s a visual guide to help:
A Beginner’s Guide to Working with JSON Files
Now that you know how to open a JSON file, let’s explore what they are and why they’re important. JSON, which stands for JavaScript Object Notation, is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. JSON is often used for web APIs, and is replacing XML as the preferred data format for web services. JSON is also used in many data storage and exchange formats, from MongoDB to Firebase.
When you open a JSON file, you’ll see a nested structure of keys and values, much like a dictionary in Python or a table in a database. This nested structure is what makes JSON so flexible and powerful. You can use tools like Python to manipulate and extract data from JSON files, which can be especially useful when working with large or complex datasets.
There are many reasons why you might want to work with JSON files. Perhaps you’re building a web application that needs to consume data from an API, or you’re conducting research that involves analyzing large datasets. Whatever your use case, JSON is a valuable skill to have in your programming toolbox.
Mastering JSON File Parsing in Python
Python is a powerful programming language that makes working with JSON files a breeze. Here’s a quick overview of how to work with JSON files in Python:
- Import the json library:
import json
- Load in your JSON file:
with open('my_file.json') as f: data = json.load(f)
- Manipulate and work with your data!
Here’s an example of how to extract a specific value from a JSON file:
with open('my_file.json') as f:
data = json.load(f)
my_value = data['my_key']
As you can see, working with JSON files in Python is very straightforward. Python’s built-in json library makes it easy to load, manipulate, and save JSON files.
7 Free Tools for Working with JSON Files
To make working with JSON files even easier, there are many free tools available online. Here are seven of the best:
- JSON Editor Online: A powerful online editor for editing, analyzing, and sharing JSON data.
- Code Beautify JSON Viewer: An online tool for formatting and analyzing JSON data.
- JSONLint: A validator and reformatter for JSON data.
- JSON Formatter: A web-based JSON formatter and validator.
- JSON Schema Generator: A free online tool for generating JSON schemas.
- JSON to CSV Converter: A handy tool for converting JSON data to CSV format.
- JSON to CSV Converter: Another useful tool for converting JSON data to CSV format.
Each of these tools has its own unique features and benefits, so be sure to explore them all to find the best one for your needs.
Tricks and Tips for Parsing Complex JSON Files
While working with JSON files is generally straightforward, there are times when you might encounter complex or nested JSON structures that can be tricky to parse. Here are some tips to help you navigate these situations:
- Use a JSON validator to make sure your JSON file is valid and well-formed
- Use Python’s built-in pprint library to format and print your JSON data in a more human-readable way
- Use Python’s built-in indexing and slicing features to extract specific values from nested JSON structures
- Take advantage of online tools and libraries like jq or jsonpath to help parse and manipulate complex JSON files
By following these tips, you’ll be able to parse even the most complex JSON files like a pro!
Common Mistakes to Avoid When Parsing JSON Files
As with any programming task, there are certain common mistakes that can trip you up when working with JSON files. Here are some of the most common mistakes to watch out for:
- Forgetting to wrap your JSON data in brackets { }
- Misusing quotation marks or other punctuation
- Missing required keys or values in your JSON structure
- Not properly closing your JSON file
- Misunderstanding the structure or contents of your JSON file
By being aware of these common mistakes, you can avoid making them yourself and become a more confident and proficient JSON file parser.
Conclusion
In this article, we’ve explored the different ways to open a JSON file, as well as the best tools and techniques for working with them. From using Python to parse and manipulate JSON data to exploring tricks and tips for dealing with complex JSON structures, we hope that this guide has given you the knowledge and skills to become a more proficient JSON file parser.