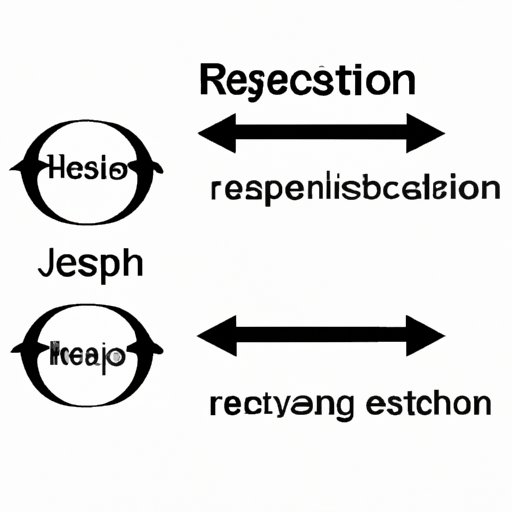
I. Introduction
If you’re working on a website or web application, you might find yourself needing to refresh a page in some scenarios. Whether you just published new code, or you want to update content, you need a way to refresh the page without disrupting a user’s experience. Fortunately, JavaScript offers several methods to refresh a page. In this article, we’ll cover five simple methods you can use to refresh a page in JavaScript.
II. The Simplest Way: Using location.reload() Method
If you need a quick way to reload a page, the location.reload()
method is probably the easiest to use. The location object in JavaScript is an interface that provides information about the current URL and allows you to navigate to a new URL. By calling the reload()
method of the location object, you can reload the current page.
Here’s an example:
location.reload();
However, keep in mind that this method has some limitations. For example, it doesn’t give you any control over how the page is reloaded, and it will refresh the entire page including all its resources and scripts. So, if you have a lot of assets and scripts, it may take some time for the page to reload completely.
III. The Refresh Button: Creating a Custom Refresh Button
Another way to refresh a page is to create a custom refresh button on your site. By using a custom button, you can control when and how the page is refreshed. This method is good if you want to provide a more user-friendly approach to refreshing the page.
Here’s how you can create a simple HTML button:
When the user clicks the button, the location.reload()
method will be called, and the page will be refreshed.
Again, keep in mind that this approach also has some limitations. The custom button may not be as intuitive for some users, and, like the previous method, it will refresh the entire page.
IV. The Timer Approach: Refreshing Pages Automatically
If you need to refresh a page automatically, you can use the setInterval()
method in JavaScript. This method allows you to execute a function at specified intervals, in this case, to refresh the page.
Here’s an example:
setInterval(function() {
location.reload();
}, 30000);
In this example, the setInterval()
method calls the location.reload()
method every 30 seconds to refresh the page automatically. This method is useful if you have a page that needs to refresh continuously, such as a news feed or a live scoreboard.
However, keep in mind that this approach has some limitations as well. The user might find the automatic refresh annoying, and some browsers may block automatic page refresh for security reasons.
V. The AJAX Approach: Refreshing Part of a Page without Reloading
If you only need to refresh part of a page, you might use AJAX, which stands for Asynchronous JavaScript and XML. AJAX enables you to asynchronously load data into a portion of a web page without reloading the entire page.
Here’s an example:
function refreshData() {
var xhr = new XMLHttpRequest();
xhr.open('GET', '/data', true);
xhr.onload = function() {
document.getElementById('data').innerHTML = xhr.responseText;
};
xhr.send();
}
setInterval(refreshData, 30000);
In this example, the XMLHttpRequest()
method requests data from the server, and the innerHTML
property updates the specified <div>
element with the received data without refreshing the entire page.
The AJAX approach is useful when you have a portion of a page that needs updating frequently, such as a chat box. It provides a smoother experience for the user as it doesn’t reload the entire page every time new content is added. However, keep in mind that AJAX has some limitations too, such as potentially slower performance, compatibility issues with older browsers, and extra complexity in its implementation compared to some of the other methods.
VI. The History Object: Refreshing Pages with the History Object
The history object in JavaScript allows you to access the browsing history of a user, including the URLs and state of each page. You can use this information to navigate through the history or refresh a page.
Here’s an example:
history.go(0);
In this example, the history.go(0)
method tells the browser to reload the current page.
The history object is useful if you need to retain some data as it reloads the page, or if you want to move back and forth between previous pages on the browsing history. However, keep in mind that some browsers may not be fully compatible with this method.
VII. Conclusion
As you can see, there are several ways to refresh a page using JavaScript. Depending on what you need to accomplish, you may choose one method over another. To summarize, the five methods we covered are:
- The
location.reload()
method - Creating a custom refresh button
- Using the
setInterval()
method to refresh pages automatically - Using AJAX to refresh part of a page without reloading the entire page
- Using the history object to refresh pages or navigate through the browsing history
Remember to test each method on your site and find out which one works best for you.