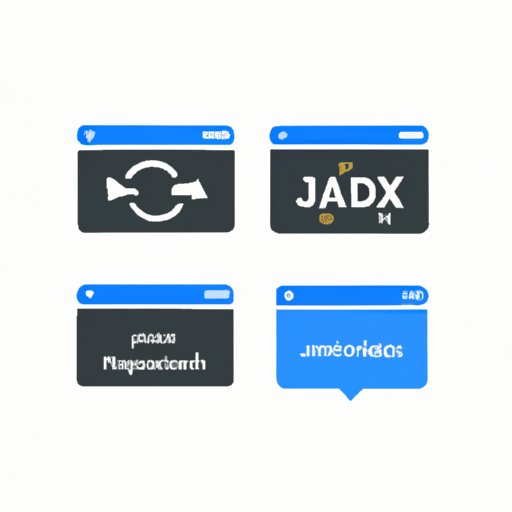
Introduction
When building web applications, it’s often necessary to reload a page to update its contents. This can be done manually using a browser’s refresh button, but as a developer, you need to know how to reload pages programmatically to expand your app’s functionalities. In this article, we’ll explore multiple methods of reloading web pages in JavaScript.
Step-by-Step Guide
The most straightforward method to reload a web page using JavaScript is to utilize the `location.reload()` method. This is a built-in method that reloads the current page, as shown below:
“`
location.reload();
“`
You can also use `location.reload(true)` to force the reload from the server instead of the cache.
If you wish to refresh a web page using a button, you may add an `onclick` attribute to the button element that calls the `location.reload()` method, as shown below:
“`
“`
Step-by-Step Instructions for Beginners on How to Refresh/Reload a Web Page with JavaScript
Here are some step-by-step instructions for beginners to practice reloading web pages using JavaScript:
1. Create a new HTML file and insert a button element with an “id” attribute set to “reload-btn”.
“`
“`
2. Create a new JavaScript file and link it to the HTML file using the `script` tag.
“`
“`
3. In the JavaScript file, select the button element with the “id” attribute of “reload-btn” using the `document.getElementById()` method and assign it to a variable.
“`
const reloadBtn = document.getElementById(“reload-btn”);
“`
4. Add an event listener to the button that listens for a click event and calls the `location.reload()` method on the current page.
“`
reloadBtn.addEventListener(“click”, () => {
location.reload();
});
“`
Screenshots for a Visual Aid
Below is a screenshot of the above code in action:

Simple JavaScript Code
While using `location.reload()` is straightforward, it does not offer fine-grained control over when and how the page is refreshed. Another option is to use JavaScript to automatically refresh the page without having to click anything.
Here’s an example of how to refresh the page automatically after five seconds using JavaScript:
“`
setTimeout(() => {
location.reload();
}, 5000);
“`
The `setTimeout()` method is used here to delay the `location.reload()` method call by five seconds.
Advantages of this Method over Traditional Reloading
Unlike manually clicking the refresh button, using JavaScript to automatically refresh a page allows you to schedule when you want the refresh to occur based on a defined time interval. This method is useful when updating content on a page from a database or API dynamically.
HTML Meta Tag
You can also use HTML meta tags to instruct browsers to refresh a page automatically after a specified period. To do this, add the following code to the `head` section of your HTML file:
“`
“`
The `content` attribute specifies the number of seconds the page should wait before refreshing.
Practical Examples of Avoiding the “Back” Button Dilemma
The HTML meta tag method is useful in avoiding the “back” button dilemma. For instance, after submitting a form on a web page, it is common to get a confirmation message with a “Thank you” note. When you click the “Back” button, the action could repeat, and another form will be submitted. However, by using the HTML meta tag with a timer, the page will automatically reload after the specified period, avoiding the issue.
Site Performance Impacts
Automatically refreshing a web page can negatively impact site performance, especially if done frequently or with a short period. Therefore, it’s recommended to use scheduling techniques such as `setTimeout()` or longer intervals to avoid performance degradation.
Keyboard Shortcuts
In addition to the reload button, most web browsers offer keyboard shortcuts to refresh a page. Here are some commonly used ones:
– F5: Reload page on all web browsers (Windows and Linux).
– Ctrl + R: Reload page on all web browsers (Windows and Linux).
– Command + R: Reload page for Safari and Chrome browsers on macOS.
– Ctrl + Shift + R: Reload page ignoring browser cache.
List of Different Shortcuts Available
Operating System | Browser | Shortcut |
---|---|---|
Windows/Linux | Chrome, Firefox, Edge, Opera, Safari | F5 or Ctrl+R |
macOS | Chrome, Firefox, Edge, Opera, Safari | Command+R |
Windows/Linux/macOS | Chrome | Ctrl+Shift+R |
Windows | Internet Explorer | F5 or Ctrl+R |
Server Side Refreshing
Another way to update content on a web page programmatically is through server-side refreshing, where the server pushes changes to the page without the need for client-side scripting.
Use Cases for Server-Side Refreshing
Server-side refreshing is useful when working with real-time web applications, such as chat applications or stock market platforms, where users require constant updates.
Step-by-Step Guide on How to Setup Server-Side Refreshing
Setting up server-side refreshing depends on the server technology in use. For example, to setup server-side refreshing using PHP, you need to use the `header()` function to set the `Cache-Control` header of the response to `’no-cache’` and `Pragma` to `’no-cache’`.
“`
“`
The above code disables browser caching and acknowledges the browser to request a new copy of the page every time.
AJAX Method
AJAX (Asynchronous JavaScript And XML) is a technique commonly used in developing dynamic web pages. AJAX allows partial refreshing of web pages without having to reload the entire page.
Explanation of Using AJAX for Page Refresh Without Reloading the Full Page
To reload a specific section of a page using AJAX, you need to define a container where the content should be updated and use the `setInterval()` function to schedule the refresh.
“`
“`
This code schedules an AJAX request for every five seconds using the `setInterval()` function. When the response is loaded, it updates the `div` with the `id` of “container”.
Sample AJAX Code
Here’s an example of an AJAX code that sends a GET request to the server and displays the response:
“`
const xhr = new XMLHttpRequest();
xhr.open(‘GET’, ‘/path/to/resource’, true);
xhr.onload = () => {
if (xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send();
“`
Using Browser Extensions
Other options for automating the process of refreshing web pages involve installing browser extensions. Here are some popular ones:
– Auto Refresh by 64 Pixels for Chrome and Firefox
– Easy Auto Refresh for Chrome
– Super Auto Refresh for Chrome
– Easy Refresh for Safari
– ReloadAll! for Opera
Description of the Features of Each Extension
Each browser extension offers different features, such as setting a custom refresh interval, reloading an entire browser window, or refreshing multiple tabs at once.
Conclusion
In this comprehensive guide, we covered seven different methods of reloading a web page using JavaScript and various technologies. Each method has its advantages and use cases, depending on the specific requirements of your web application. We encourage you to try each method and find the one that best suits your needs. Remember, effective reloading of web pages will improve user experience and create dynamic, real-time interactions on your web application.