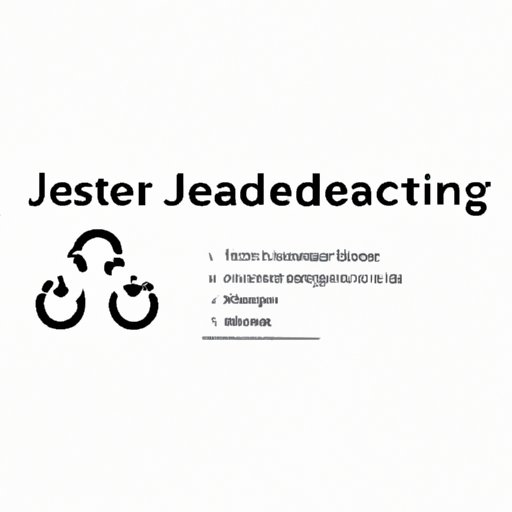
Introduction
Reloading a page is a common task in JavaScript. It may be necessary to update the contents of a page or reset the user’s session. In this article, we will explore various methods to reload a page in JavaScript.
Using location.reload() method in JavaScript
The location.reload() method reloads the current page. This method can be used when you want to force a page reload without caching the page.
Here’s an example of how to use the location.reload() method:
“`
location.reload();
“`
Use this method when you need to reload the page and do not want to cache it.
Using the reload() method of the location object in JavaScript
The reload() method of the location object is similar to the location.reload() method. It reloads the current page without caching it.
Here’s an example of how to use the reload() method:
“`
location.reload(true);
“`
Use this method when you need to reload the page and do not want to cache it.
Reloading the page using the window object in JavaScript
The window object represents an open window in a browser. It has properties and methods to manipulate the window.
To reload the page using the window object, use the location.reload() method:
“`
window.location.reload();
“`
Use this method when you need to reload the page and do not want to cache it.
Using location.href or location.replace in JavaScript to reload the page
The location.href and location.replace properties of the location object can be used to load a new document or replace the current one, respectively.
To reload the page using location.href, use the following code:
“`
location.href = location.href;
“`
To reload the page using location.replace, use the following code:
“`
location.replace(location.href);
“`
Using location.href or location.replace may cause multiple requests to the server, which can increase server load. Use with caution.
Implementing a button on the page which calls the location.reload() method when clicked
To implement a button on the page which calls the location.reload() method when clicked, you will need to create a button and add an event listener to it.
Here’s an example of how to create a button in HTML:
“`
“`
Here’s an example of how to add an event listener to the button:
“`
document.getElementById(‘reload-button’).addEventListener(‘click’, function() {
location.reload();
});
“`
This code selects the button using the getElementById method and adds an event listener to it. When the button is clicked, the location.reload() method is called.
Using meta tags to auto-refresh the page at a specified interval
Meta tags are HTML tags that provide metadata about a document. They can be used to specify page description, keywords, and auto-refresh rate, among others.
To auto-refresh the page at a specified interval, use the following meta tag:
“`
“`
This code specifies that the page should be refreshed every 30 seconds.
Conclusion
Reloading a page is a common task in JavaScript. In this article, we explored various methods to reload a page in JavaScript, including using the location.reload() and reload() methods, window object, location.href and location.replace properties, adding a button, and using meta tags for auto-refresh. Try out these methods in your JavaScript projects and choose the most appropriate one for your needs.