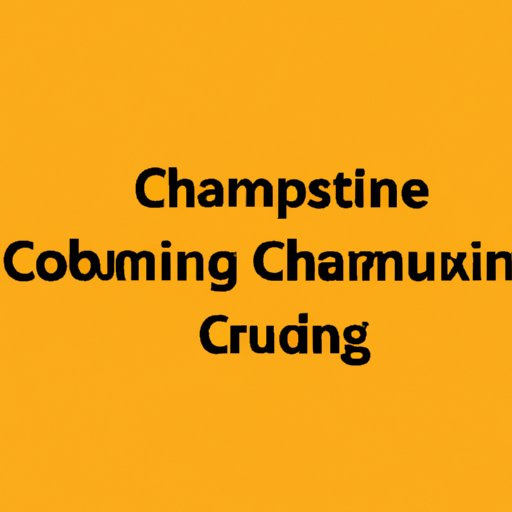
I. Introduction
Running C programs can be a challenging task, especially for beginners who are just starting to learn the language. Common problems when running C programs include syntax errors, compiler issues, and debugging difficulties. However, being proficient in running C programs is essential for anyone who wants to develop software applications, system utilities, device drivers, or embedded systems.
In this guide, we will explore the best practices for running C programs like a pro. We will provide you with tips, techniques, and strategies for debugging, troubleshooting, and optimizing your code. Whether you are a beginner or an experienced programmer, this guide will help you enhance your skills and become more proficient in running C programs.
II. 7 Tips for Running C Programs Like a Pro
Here are seven tips for running C programs like a pro:
Use the right compiler
Choosing the right compiler is essential for running C programs. The compiler is software that translates your C code into machine language that the computer can understand. There are several compilers available, including GCC, Clang, Visual Studio, and Turbo C++. Make sure to select a compiler that is compatible with your operating system and hardware architecture.
Pay attention to syntax errors
Syntax errors are errors in the structure of your code. They can cause your program to fail to compile or run correctly. Make sure to pay attention to syntax errors by using a syntax highlighter and running your code through a syntax checker.
Debugging tips
Debugging is the process of finding and fixing errors in your code. There are several debugging techniques you can use, including print statements, step-by-step debugging, and memory allocation tracking. Make sure to use a debugger that is compatible with your programming environment.
Get familiar with command line tools
Command line tools are programs that you can run from the command prompt. Command line tools are useful for compiling, linking, and running C programs. Some popular command line tools include gcc, gdb, and make. Make sure to learn how to use command line tools to become more proficient in running C programs.
Use version control systems
Version control systems are software tools that help you keep track of changes in your code. They allow you to save different versions of your code and collaborate with other developers. Some popular version control systems include Git, SVN, and Mercurial. Make sure to use a version control system to keep track of your code changes.
Optimize your code
Code optimization is the process of improving the performance of your code. There are several techniques you can use to optimize your code, including loop unrolling, inlining, and function specialization. Make sure to optimize your code to improve its performance and reduce its memory usage.
Document your code
Documenting your code is essential for maintaining its readability and accessibility. Make sure to use comments, annotations, and documentation tools to document your code. By documenting your code, you can improve its maintainability and understandability.
III. The Ultimate Guide to Running C on Your Machine
If you are new to running C programs, you might wonder what tools and software you need on your machine. In this section, we will guide you through the process of setting up a development environment and running your first C program.
System requirements for running C programs
To run C programs on your machine, you need a compiler, a text editor, and a command prompt. Make sure that your machine meets the system requirements for running C programs.
Installing a C compiler on Windows/Mac/Linux
There are several compilers available for running C programs on different operating systems. To install a C compiler on your machine, you can follow these steps:
- Choose a compiler that is compatible with your operating system and hardware architecture.
- Download the compiler from the official website or a trusted source.
- Install the compiler by following the instructions provided in the installation wizard.
Setting up a development environment
Before you can start running C programs, you need to set up a development environment that includes a text editor, a compiler, and a command prompt. Some popular development environments for C programming include Visual Studio Code, Eclipse, and Code::Blocks.
Hello World program tutorial
The Hello World program is a simple C program that displays the message “Hello, World!” on the console. It is often used as a starting point for learning C programming. Here is a sample code for the Hello World program:
“`
#include
int main()
{
printf(“Hello, World!\n”);
return 0;
}
“`
To run the Hello World program, you can follow these steps:
- Open a text editor and type the Hello World code.
- Save the code as helloworld.c.
- Open a command prompt and navigate to the directory where you saved the code.
- Compile the code by typing “gcc helloworld.c -o helloworld”.
- Run the program by typing “helloworld”.
IV. Master the Art of Running C Programs with These Simple Steps
Running C programs requires a certain set of skills and techniques. In this section, we will show you how to master the art of running C programs by structuring your code, using preprocessor directives, and organizing your work.
Basic workflow of running C programs
The basic workflow of running C programs involves the following steps:
- Write your C code in a text editor.
- Compile the code using a compiler.
- Link the object files using a linker.
- Run the executable file.
Structuring your code for readability
Structuring your code is essential for improving its readability and maintainability. You can structure your code by breaking it down into functions, using meaningful variable names, and organizing your code into modules. By structuring your code, you can make it easier to understand and modify.
Using preprocessor directives
Preprocessor directives are statements that are executed before the code is compiled. They are used to define constants, include header files, and perform conditional compilation. Some popular preprocessor directives include #define, #include, and #ifdef. By using preprocessor directives, you can improve the efficiency and flexibility of your code.
Tips for code organization
Code organization is essential for managing complexity and improving code quality. Some tips for code organization include grouping related functions and variables into modules, using encapsulation to hide implementation details, and following standardized coding conventions. By organizing your code, you can make it easier to navigate and understand.
V. Troubleshooting Common Issues When Running C: A Beginner’s Guide
When running C programs, you might encounter several common issues, including syntax errors, runtime errors, and logical errors. In this section, we will provide you with a beginner’s guide to troubleshooting common issues when running C programs.
Common errors when running C programs
Some common errors when running C programs include syntax errors, segmentation faults, and buffer overflow errors. These errors can be caused by incorrect syntax, memory allocation issues, and logic errors. By understanding these errors and their causes, you can troubleshoot them more effectively.
Debugging techniques and tools
Debugging is the process of finding and fixing errors in your code. There are several debugging techniques and tools you can use, including print statements, step-by-step debugging, and memory leak detection. Make sure to use a debugger that is compatible with your programming environment.
Working with error messages and warnings
Error messages and warnings can provide valuable information about the issues in your code. Make sure to read and understand error messages and warnings, and use them to identify the sources of the errors. By fixing the errors and warnings, you can improve the quality and robustness of your code.
VI. Streamline Your Workflow: How to Efficiently Run C Code
Efficiently running C code requires a streamlined workflow that minimizes repetitive tasks and maximizes productivity. In this section, we will show you how to streamline your workflow by organizing your work, automating build processes, and using Makefiles.
Organizing your workflow
Organizing your workflow is essential for maximizing your productivity and minimizing errors. Some tips for organizing your workflow include using version control systems, setting up a development environment, and following coding conventions and standards. By organizing your workflow, you can make your work more efficient and effective.
Automating build processes
Automating build processes involves using scripts and tools to automate repetitive tasks, such as compiling, linking, and testing your code. Some popular build automation tools include Ant, Maven, and Grunt. By automating build processes, you can minimize errors and improve the efficiency of your work.
Using Makefiles for compiling multiple files
Makefiles are powerful tools for managing complex C projects that involve multiple files and dependencies. Makefiles are used to define rules for compiling, linking, and testing your code. By using Makefiles, you can automate the build process and improve the maintainability of your code.
VII. Boost Your Productivity with These Time-Saving Methods for Running C Programs
Boosting your productivity involves using time-saving methods and techniques that make your work more efficient and effective. In this section, we will show you how to boost your productivity by using code templates and snippets, using libraries, and writing efficient code.
Code templates and snippets
Code templates and snippets are pre-existing pieces of code that can be reused for common tasks and functions. Code templates and snippets can save you time and effort by reducing repetitive coding tasks and minimizing errors. Some popular code template and snippet libraries include GitHub Gist, CodePen, and JSFiddle.
Using libraries
Libraries are collections of pre-existing code and functions that can be used to perform specific tasks and operations. Libraries can save you time and effort by providing you with pre-existing and tested code. Some popular libraries for C programming include the Standard C Library, the POSIX Library, and the GNU Scientific Library.
Writing efficient code
Writing efficient code involves following best practices and guidelines that improve the performance and memory usage of your code. Some tips for writing efficient code include using loops instead of recursion, avoiding unnecessary memory allocations, and minimizing code redundancy. By writing efficient code, you can improve the performance and scalability of your applications.
VIII. Expert Strategies: How to Optimize Your C Program’s Performance
Optimizing your C program’s performance involves using advanced techniques and strategies to improve its speed and memory usage. In this section, we will show you how to optimize your C program’s performance by using profiling tools, optimizing code speed, and memory usage.
Profiling tools for performance optimization
Profiling tools are software utilities that analyze the performance and resource usage of your code. They provide you with valuable information about the execution time, memory usage, and CPU usage of your code. Some popular profiling tools for C programming include Gprof, Valgrind, and DTrace.
Tips for optimizing code speed and memory usage
Optimizing code speed and memory usage involves using advanced techniques and strategies, such as loop unrolling, cache optimization, and function specialization. By optimizing your code, you can improve the performance and resource usage of your applications.
IX. Conclusion
Running C programs can be a challenging task, but by following the tips, techniques, and strategies we have provided in this guide, you can become a proficient C programmer. We have shown you how to set up a development environment, troubleshoot common issues, streamline your workflow, and optimize your code. Remember to document your code and use version control systems to improve the maintainability of your code. With practice and diligence, you can become an expert C programmer.