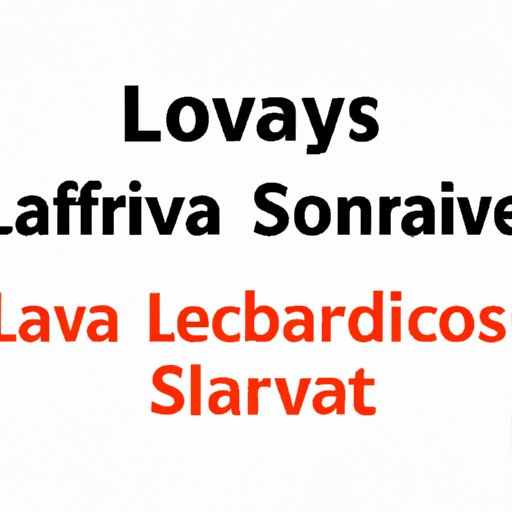
I. Introduction
Sorting is a common task in the world of programming, and Java is no different. Sorting data helps in quickly finding an item through searches by allowing developers to access information alphabetically, numerically, or in other formats. This article aims to guide you on how to sort lists in Java, the importance of sorting lists, and various techniques that will help you choose the right approach for your project.
II. Sorting a List using Built-in Tools in Java
The Collections.sort()
method is a built-in way to sort lists in Java. It is easy to use, and you can sort a list with just one line of code, as shown below:
“`java
List
Collections.sort(names);
“`
However, the Collections.sort() method has its limitations. For instance, it can only sort lists in ascending order since there is no way of defining a custom sorting order. Also, it can only sort lists that implement the java.util.List interface.
Despite these limitations, the Collections.sort() method is the preferred method of sorting in Java since it is straightforward to implement.
III. Alternative Ways to Sort a List in Java
Java provides two interfaces: the Comparable and the Comparator interfaces that allow developers to sort lists with custom sorting requirements.
The Comparable interface provides a natural ordering of objects based on the implementation of the compareTo() method in the class of the object being sorted. In contrast, the Comparator interface provides multiple sorting options for the same data type.
The following code demonstrates how you can implement the Comparable interface to sort a list of objects:
“`java
class Person implements Comparable
private String name;
public int compareTo(Person otherPerson){
return name.compareTo(otherPerson.name);
}
}
List
Collections.sort(persons);
“`
In contrast, the Comparator interface compares objects based on fields not internal to the object. Below is an example of sorting employees based on their salaries:
“`java
class EmployeeSalaryComparator implements Comparator
public int compare(Employee e1, Employee e2) {
return e1.getSalary().compareTo(e2.getSalary());
}
}
List
Collections.sort(employees,new EmployeeSalaryComparator());
“`
One benefit of implementing these interfaces is that they allow you to sort custom objects using specific fields.
IV. Comparison of Different Sorting Algorithms in Java
Java offers various sorting algorithms that you can use to sort lists. The most commonly used algorithms are QuickSort, Merge Sort, and Selection Sort.
Algorithms differ in complexity, which can have a significant impact on performance. QuickSort is one of the most efficient algorithms available, with a time complexity of O(n log n), while Merge Sort is slower and has a time complexity of O(n log n). On the other hand, Selection Sort has a higher time complexity and is more suitable for small lists.
You can implement any of these algorithms using the Collections.sort() method or the Arrays.sort() method. Below is a quick code example of how to use the quicksort algorithm to sort a list of integers:
“`java
List
numbers.add(1);
numbers.add(5);
numbers.add(2);
numbers.add(4);
Collections.sort(numbers);
“`
V. Best Practices for Sorting a List in Java
Sorting lists in Java comes with some challenges, and some practices help avoid potential pitfalls. For instance, when sorting lists that have null values, it would be best to remove them before sorting. Handling edge cases, such as sorting lists that have duplicates or those with a single item, requires attention as well.
Other best practices include optimizing the sorting process for large lists, which involves using the most efficient sorting algorithm available. Another approach is using parallel sorting algorithms to distribute sorting over multiple processors.
The following code illustrates using the java.util.concurrent package to parallelize the sorting process, which can improve performance on larger lists:
“`java
List
//populate the list with values
numbers.parallelStream().sorted().collect(Collectors.toList());
“`
VI. Using Lambda Expressions to Sort Lists in Java 8 and Higher Versions
Lambda expressions eliminate the need to implement boilerplate code when performing simple sorts. They make code shorter and easier to read.
The following code demonstrates using a lambda expression to sort a list of strings alphabetically:
“`java
List
//add names to the list
names.sort((a, b) -> a.compareTo(b));
“`
The above code sorts the list of names in ascending order.
VII. Sorting Lists with Different Data Types in Java
Sorting lists that have different data types require a custom implementation of the Comparator interface. The following code illustrates sorting a list of objects based on a specific field:
“`java
class EmployeeNameComparator implements Comparator
public int compare(Employee e1, Employee e2) {
return e1.getName().compareTo(e2.getName());
}
}
List
Collections.sort(employees,new EmployeeNameComparator());
“`
In this example, we sort employees based on their names.
VIII. Third-Party Libraries for Sorting Lists in Java
The Apache Commons library and Google’s Guava provide extra functionalities besides the built-in Java tools to sort lists. You can use Apache Commons to sort custom objects based on properties, while Guava provides an easy way to perform natural sorts.
The following code illustrates sorting a list of custom objects using Apache Commons:
“`java
List
//populate the list
Comparator
Collections.sort(employees,salaryComparator);
“`
In this example, we sort employees based on their salaries using the comparing() method of the Comparator interface.
IX. Conclusion
In conclusion, sorting lists is a crucial task for every Java developer. This article explored various techniques for sorting lists in Java, including built-in methods, alternative ways like using Comparables and Comparators, sorting algorithms, lambda expressions, and third-party libraries. We also provided best practices for sorting lists and various code examples to illustrate the techniques discussed.
We hope this article provided you with enough information to sort lists in Java comfortably. Remember, choosing the right sorting technique depends on your project’s needs. We encourage you to practice implementing these techniques in your code and using them for your real-world projects.